Exploring the “New” and “Make” in Go: Features and Uses
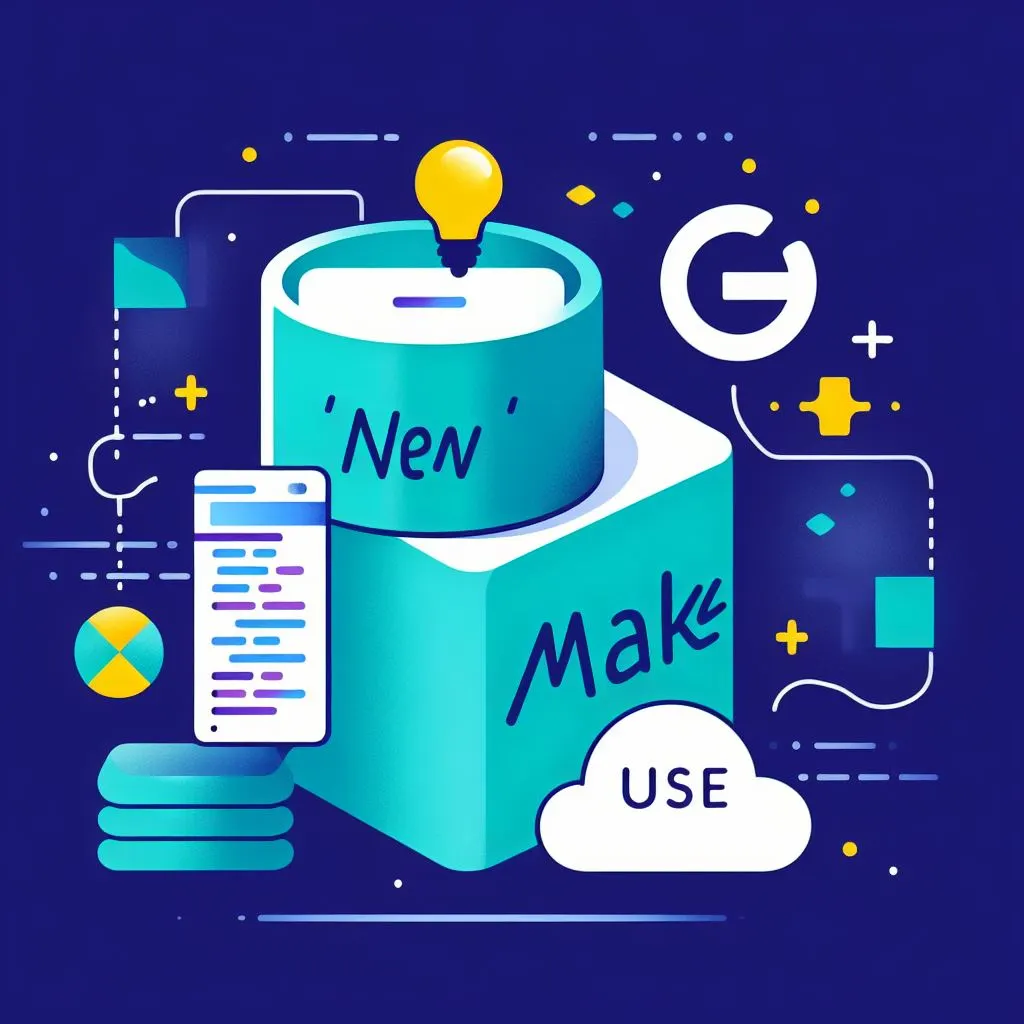
Go, also known as Golang, is a statically typed, compiled programming language developed by Google. It has gained immense popularity in recent years, thanks to its simplicity, efficiency, and robustness. In this blog post, we will delve into two essential concepts in Go: new and make. These built-in functions play a crucial role in memory […]
Synchronizing Concurrent Code with `sync.Mutex` in Go
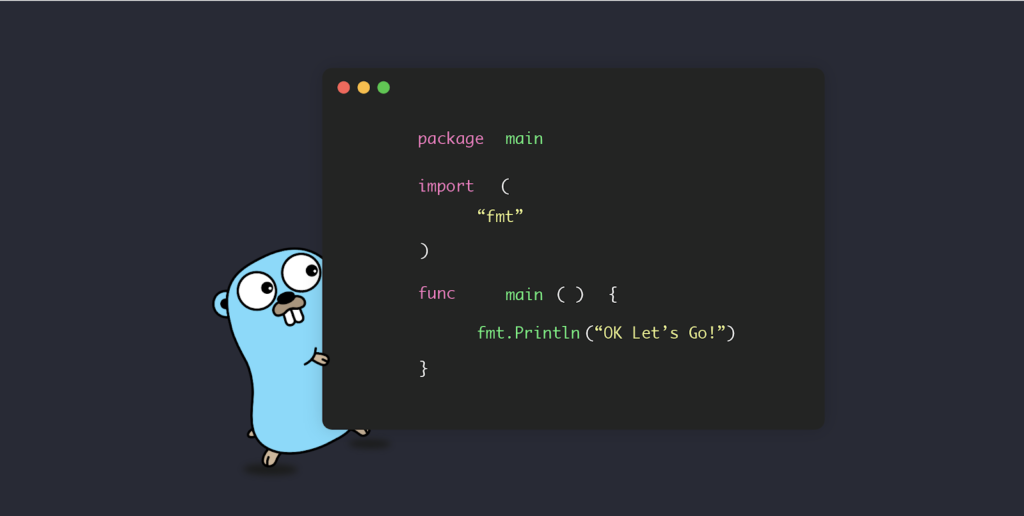
Concurrency is one of Go’s most compelling features, allowing developers to write efficient, parallelized programs. However, with great power comes great responsibility. Writing concurrent code can be tricky, as it often involves shared resources that multiple goroutines may access simultaneously. To ensure data integrity and avoid data races, Go provides synchronization primitives, including the sync.Mutex. […]
Mastering Concurrency with the Select Statement in Go
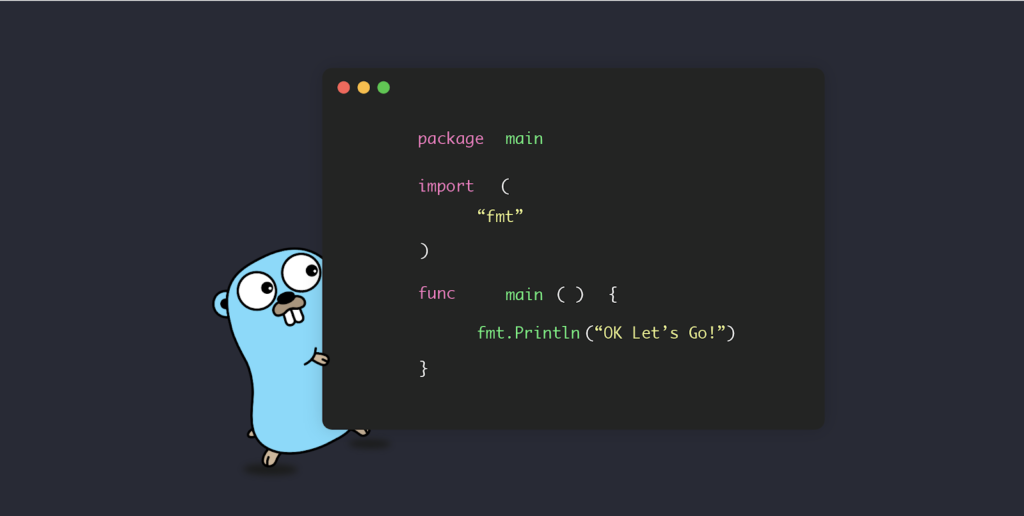
Concurrency is at the core of the Go programming language’s design, and it provides various tools to help you build efficient and responsive concurrent programs. One of these tools is the select statement, a powerful construct that allows you to work with multiple channels concurrently. In this blog, we’ll explore the select statement in Go, […]
Navigating Channels with Range and Close in Go
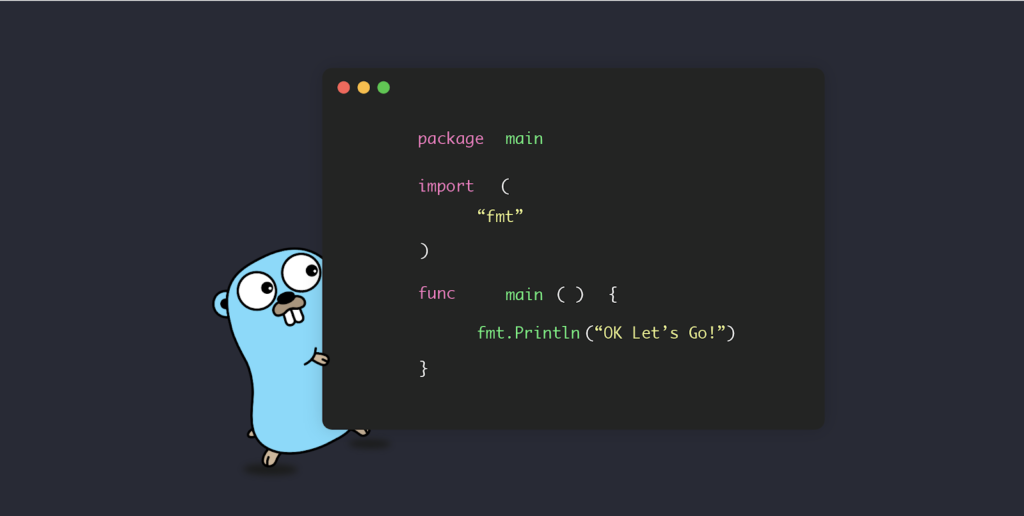
In the world of concurrent programming, Go shines with its built-in support for channels. Channels provide a powerful mechanism for communication and synchronization between goroutines. Two essential operations for working with channels are range and close. In this blog, we’ll delve into how range and close are used with channels in Go, their syntax, use […]
Buffered Channels in Go: Balancing Concurrency and Communication
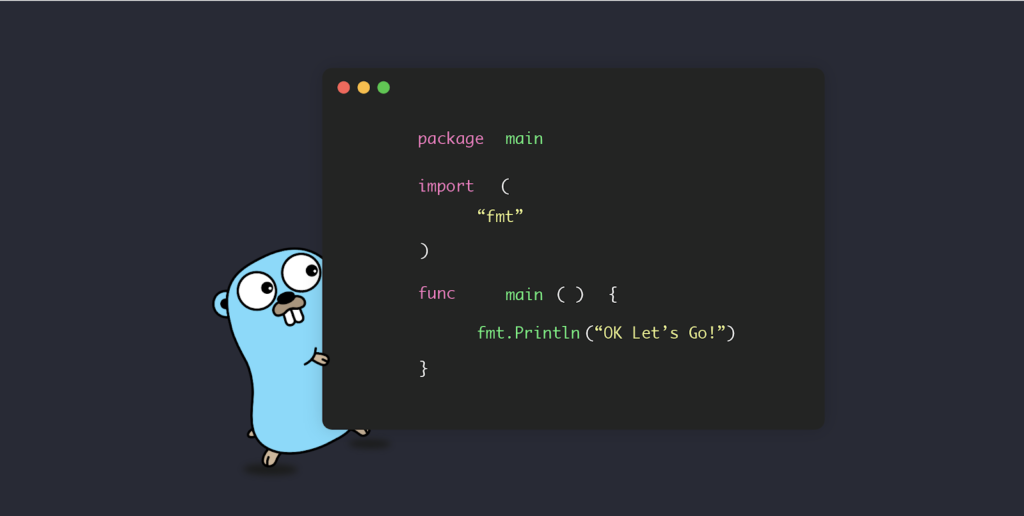
Concurrency is one of the cornerstones of the Go programming language, and channels are a fundamental tool for coordinating and communicating between concurrent goroutines. In Go, channels can be used in various ways to facilitate communication, but one important feature that enhances their capabilities is buffering. Buffered channels provide a mechanism to balance the interplay […]
Channeling Concurrency: A Guide to Go Channels and Their Use Cases
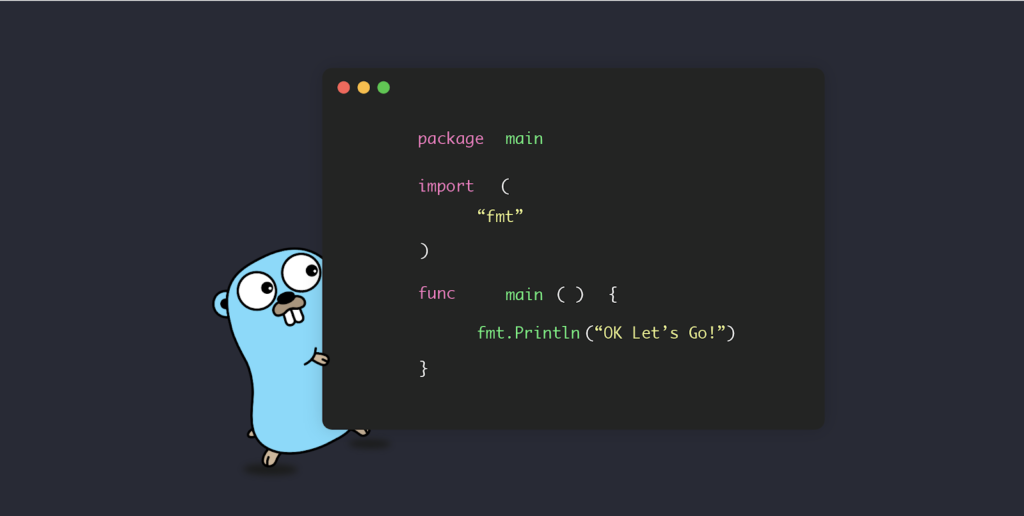
Concurrency is a fundamental aspect of modern software development, enabling programs to perform multiple tasks simultaneously. In Go, channels are a powerful and elegant way to manage concurrent communication between goroutines. In this blog, we’ll explore channels in Go, their definition, use cases, syntax, advantages, and best practices. Whether you’re new to Go or a […]
Goroutines in Go: A Concurrent Revolution
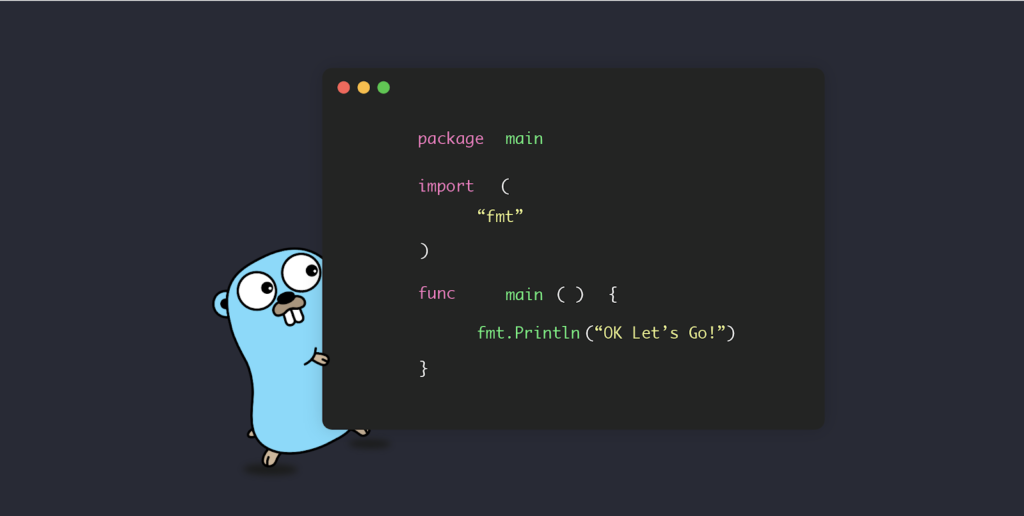
Concurrency is a crucial aspect of modern software development, enabling programs to efficiently perform multiple tasks concurrently. In the Go programming language, goroutines are at the heart of concurrency. They provide a lightweight and efficient way to run concurrent code, making it easier to develop high-performance applications. In this blog, we’ll explore goroutines in Go, […]
Unleashing the Power of Generic Types in Go
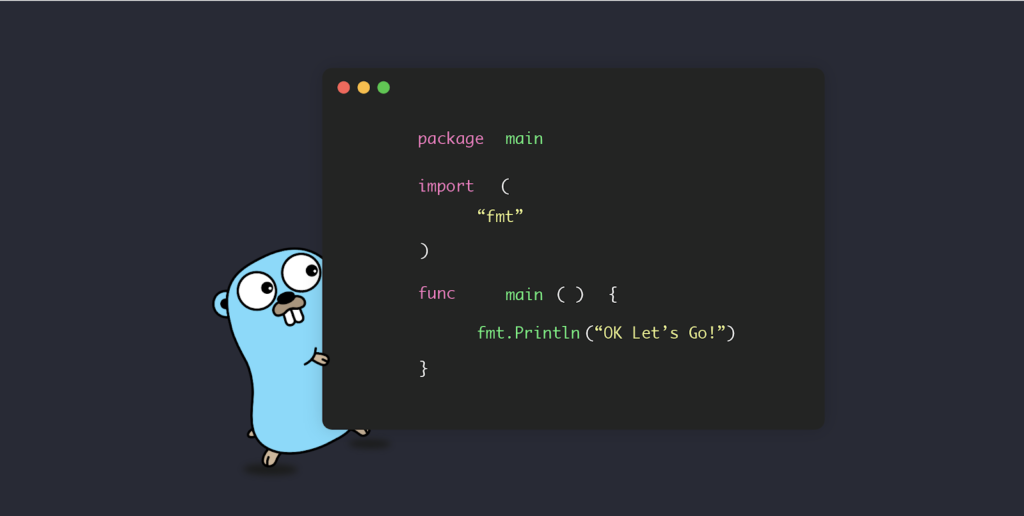
The inclusion of generic types in Go 1.18 and later versions has ushered in a new era of flexibility and code reusability for the language. Generic types allow you to write functions and data structures that work with a wide range of data types while preserving type safety. In this blog, we’ll explore the world […]
Type Parameters in Go: A Leap Towards Generics
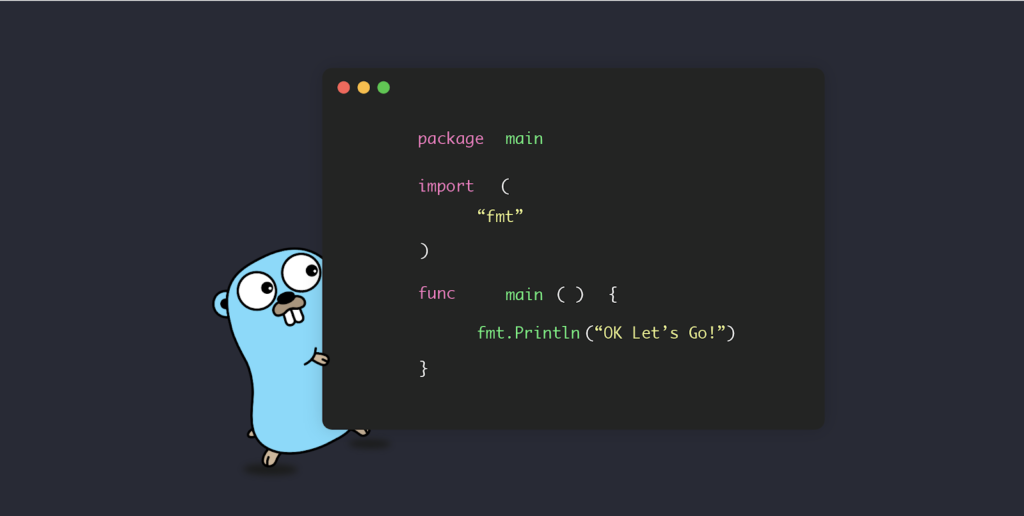
The introduction of type parameters in Go, often referred to as generics, marks a significant milestone in the language’s evolution. Generics enhance Go’s flexibility and allow developers to write more reusable and efficient code. In this blog, we’ll dive deep into the world of type parameters in Go, exploring their definition, use cases, syntax, advantages, […]
The Art of Go: Exploring the Image Interface for Graphics Manipulation
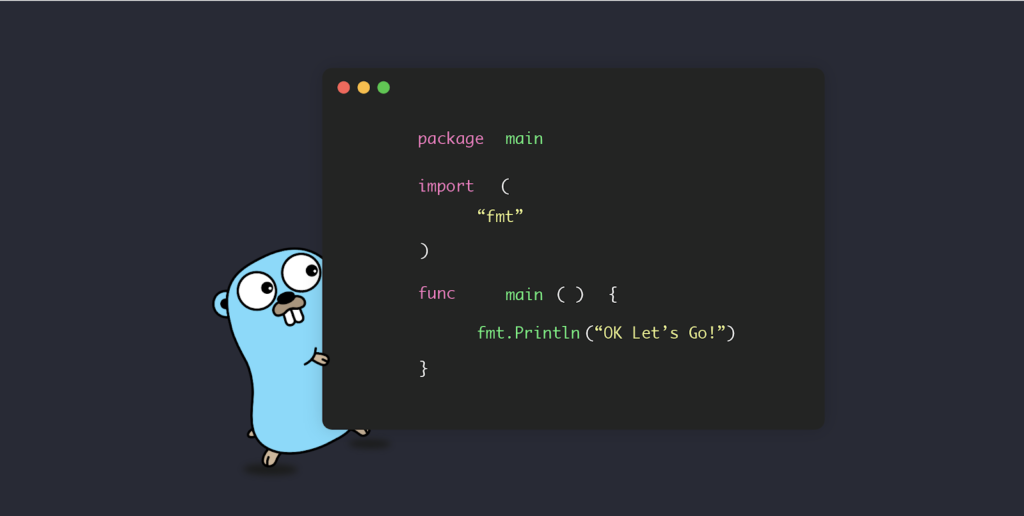
In the realm of Go programming, the image package stands as a versatile tool for working with graphics and images. At the heart of this package is the Image interface, a fundamental component that allows you to manipulate and create images programmatically. In this blog, we’ll delve into the world of the Image interface in […]